‘C’ is a language for computers, and because it programs a computer, it is known as a programming language.
Before you understand about the ‘C’ programming language in general, you need to know why is it called as a language at the first place.
Why ‘C’ is a Language?
The way by which we humans communicate with each other, is known as a language.
If you communicate by symbols or signs, it called as a sign language which does not involve any verbal or words.
Otherwise, we have many word based language like English, Hindi, Portugese etc.
Similarly, C is language that we use to communicate with a computer.
A computer does not have it’s own intelligence. We have programmed a computer with several instructions and that is how a computer runs the instructions all the time in the same order.
When a computer powers up, it has a BIOS program that is written to test all the input/output (I/O) devices, runs every time in the same sequence.
Because C is used to communicate with a computer it is known as a language and as it is used to program a computer it is known as a programming language.
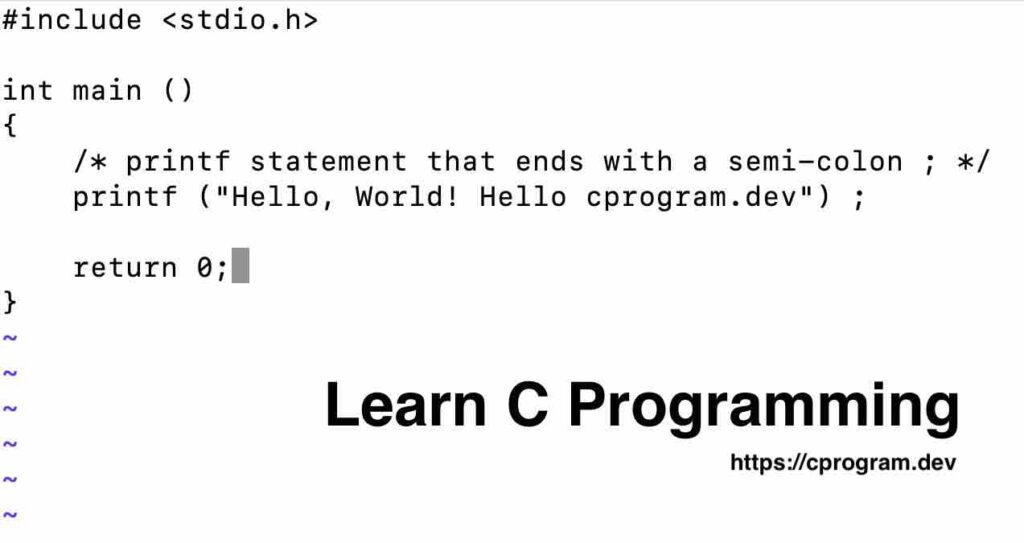
What is a C Program?
a small piece of code written in C language is known as a C Program. The first easiest one is a Hello World program in C which is as below:
1. #include <stdio.h>
2.
3. main ()
4. {
5. /* Prints Hello,World! on the screen. */
6. printf ("Hello, World!");
7. }
Code language: PHP (php)
Here is the explanation of the C program line by line.
1st line is a supporting line for the printf() function added in line number 6. With the help of 1st line the C compiler knows what does the printf() means.
3rd line tells that this is where our program starts. Be default any C program starts with a main() function.
4th and 7th line are the actual function start and end. Any function in C language starts with a ‘{‘ curly brace and end with a ‘}’ curly brace.
5th line is just a comment to be readable by humans. Any statement which is kept inside /* */ will be ignored by a C compiler
6th line is a printf () function which has a very specific type. ptintf (“”) ; Anything you put inside the double quote of a printf statement will get printed on the screen. Observe the ‘;’ semi colon at the end of the line which tells the C compiler that this statement ends here.
This is the end of the explanation.
Of course there are so many new things for you to understand and grasp from this small C program but don’t worry, this is not the end, it is just the beginning.
Stay with me on this site and I will explain more and more about C Programs, and C Programming Language in detail in other posts.
Now to make it a little more simple for you, I will take you through the building blocks of C Programing Language.
Why C Program was Invented?
Before you learn C Progamming Language you must know why was this invented at the first place.
C Language was invented around the 1970s and this was not the first programming language that was developed.
Several other programming languages such as Algol, Fortran, B Language etc. were invented before the C Language.
Going back to history of each programming language and how they evolved is not required. But one thing needs to be remembered, why all these languages are evolved at the first place?
The answer is to make it more human readable.
What does human readable means here?
To make you understand what that is, I need to explain about the low level programming and how hard that was for humans to read and understand.
Understanding Binary and the Relation to Programming
It all starts with Electronics (hardware), and not the software. There is no software without a hardware.
The invention of Flip-Flops, registers etc, which are basically Electronics devices and are the building blocks of any hardware, lead to storage, logic etc.
Flip-flop, Register etc. work on the principle of 0 and 1. A high voltage that represents 1 and a low voltage represents 0. This principle is used to expand the logic on software.
If you don’t know, computing started from binary numbers 0 and 1, that’s it. Over the period of time, these small 0s and 1s have been used to construct more bigger things.
We can write any numeric digit by combining just 0 and 1. If you do not know, please read binary language a little bit to get to the history here. It is important.
The number system that we learn from our childhood is known as decimal numbers. Similarly, the number system that comprises of 0 and 1 is known as binary number system.
And there is a different number system that consists of numbers and alphabets which is known as Hexadecimal number system.
Let’s look at the below conversions:
Decimal number | Hexadecimal Number | Binary number |
0 | 0 | 0 |
1 | 1 | 1 |
2 | 2 | 10 |
3 | 3 | 11 |
4 | 4 | 100 |
5 | 5 | 101 |
6 | 6 | 110 |
7 | 7 | 111 |
8 | 8 | 1000 |
9 | 9 | 1001 |
10 | A | 1010 |
13 | D | 1101 |
14 | E | 1110 |
15 | F | 1111 |
16 | 10 | 10000 |
If you see the first number is of a single binary digit and the number ten is consists of 4 binary digits.
Binary digit in short called a Bit. If take a closer look at the pattern, to represent a decimal number it requires 2n. Keep in mind that in binary we start counting from 0. So, to represent the 8th number in binary which is 7 in decimal requires 3 bits (binary digits) 111.
So, with the help of a combination of 4-bits I can complete the basic Hexadecimal numbers.
Now these Hexadecimal and Binary numbers are use to write machine instructions which is generally used in Assembly language.
Assembly language is one of the early human readable language. We can convert any Assembly code into binary values without any more intermediate conversion, hence this was called as a low level language because it was close to the machine code which is binary.
Microprocessor vendors like Intel, will release the instruction set that is supported by a microprocessor like 8085. These instruction sets are based on Hex values which will be converted by an assembler to binary code.
Some sample assembly code is as below:
main: push %rbp # establish...
mov %rsp, %rbp # ...a stack frame (and align rsp to 16 bytes)
lea str(%rip), %rdi # load the effective address of str to rdi
xor %al, %al # tell printf we have no floating point args
call printf # call printf(str)
leave # tear down the stack frame
ret # return
Code language: PHP (php)
Slowly the research and development on programming languages evolved and we got many other high level programs which were more human readable than the low level languages like this Assembly language.
C programming language is one of the first High Level language that comprises of terms like: do, while, for, int, char, etc which made programming simpler as compared to the previous ones.
Even now, all the C code is converted into assembly code which can still be read by decoding a compiled binary file using utilities like Objdump, Readelf etc.
Building Blocks of C Language
Once you understood the basics of Electronics and how a programming language came into this world, let’s take a look straight into the building blocks of C language.
To make it easier for you to start understanding C, let’s look at C as a language and its building blocks.
For this, I will take English as a language to compare C Language.
English Language vs C Language
It is important to understand this part so that you can get started with C Programming Language very easily.
- What are the building blocks of English? The Alphabets, A – to – Z.
- First you understand these letters, how do they sound, etc.
- Exactly same in C, the Keywords are the Alphabets of C. I have added more details on this in a next section.
- Like the way alphabets are the building blocks in English but they are meaningless when used alone (except few words which you can ignore for the sake of understanding).
- So, how do we use Alphabets? We use alphabets to make words and these words makes a complete statement. That’s what the true usage of Alphabets.
- Similarly, we use Keywords to create meaningful declarations or statements in C.
- How to know when a statement started? We all know that a statement in English starts with a capital or upper case letter.
- Similarly, a C program, starts with a main () function.
- Anything you write on the main () function will be executed in top-down order, remember this.
- Now, how to know when a statement ends? With a full stop or period ‘.’ .
- Exactly the same way a statement in C ends with a semi-colon ‘;’ .
- The way we have many more rules to make statements in English, exactly same way we have many more rules to use these Keywords to create functions, structures etc. which we will look when we go deep into the specific topics.
To understand the basics of C, I will stick with Keywords, variable declarations and statements.
Keywords in C
Keywords are the Alphabets of C language.
Below are the keywords available in C which corresponds to a specific meaning as well, which I have written next to it.
Keyword | Usage |
int | used to declare an integer value Declaration statement: int house_number; Here, house_number is an integer variable and can hold only values from -n – 0 – +n |
char | used to declare a single character value Declaration statement: char section; section is a character type value which holds only a single character which may vary from A – Z or a – z, or any other ASCII value. |
float | Used to declare a decimal value. Declaration statement: char section; |
if | |
else | |
for |
To keep it simple for you, I have not included all the Keywords available in C. I have added only a very few keywords which will be easier for you to understand when you are starting for the first time.
mian () function in C
To make it a standard practice, every C program starts with a main () function only.
Whatever code you write inside that function will be executed in the order they are written.
If you write any executable statement outside main function, that will be discarded or a compilation ERROR will thrown at you.
You cannot add an executable statement but you can declare any variable outside main() function. We will look at more detailed things in relevant topics.
Statement in C
How do you want to start with a C program?
If you just want to print some text, let’s take the example of the Hello, World C program:
#include <stdio.h>
main ()
{
/* printf statement that ends with a semi-colon ; */
printf ("Hello, World!") ;
}
Code language: PHP (php)
Here, the printf ("Hello, World!");
is a statement and it ends with a semi-colon ‘;’ .
Variable declaration and Assignment
If you want to deal with number, then the sample program would look something like this:
#include <stdio.h>
main ()
{
int number_variable;
number_variable = 5;
/* printf statement that ends with a semi-colon ; */
printf ("Value of the integer number_variable is %d", number_variable) ;
}
Code language: PHP (php)
In this program, I have declared an integer variable of name number_variable.int number_variable;
I have assigned that variable, a number of 5number_variable = 5;
As both of them are also statements, they also end with a semi-colon ‘;’ .
You can also see that the printf statement contains a %d and then the variable name after a comma. This is how the printf () function works.
For now, you focus only on the variable and assignment value to that. I will explain everything in detail in other relevant topics.
Running your C Program Code
This is the most fun part.
Once you have written your own first C program, it is time to compile and run it.
C Program Compilation
Compilation is the process of converting human readable code to machine understandable code.
If you remember the section of understanding Binary and its relation to C Program, I have explained that 1s and 0s are the numbers which is understood by the computer.
So, the C program written by you in mere English language is not directly understood by the processor of your computer.
This is where a C compiler takes your program, modifies it to low level assembly language or machine readable language which can be executed buy the processor.
Compile C Program in Linux
By default most of the Linux OS distributions like Ubuntu, Linux Mint, etc. comes with the gcc compiler installed in it.
So, you just execute the below command to compile your c program, assuming the filename is first-program.c:
$ gcc first-program.c
After executing the above command, this will generate two file, first-program.o and a.out.
Compile C Program on Mac
Just like Linux, MacOS also comes with the gcc compiler installed with it.
Compile the c code and rest everything stays similar to
$ gcc first-program.c
After executing the above command, this will generate only one file contrary to Linux, which is the a.out.
Compile C Program on Windows
By default Tthere is no application that provides Gcc compiler on Windows. You need to install any one IDE (Integrated Development Environment) application which has a gcc in it.
Some examples are: CodeBlocks, Eclipse, Visual Studio etc.
After installing the IDE, open it, you create your C program using that and you can compile and run from that IDE itself.
Run the C Program
Once you have compiled your C program and you have got the a.out file, just run that file will show the program output.
$ ./a.out
When I executed the Hello, World program, I got the below output on my Mac:
sibsahu@SIBSAHU-M-C6JD C-program % ./a.out
Hello, World!
Conclusion
Understanding C Program is not a days job. First it needs an initiation from your side and then you need to be consistent in practicing different types of programs.
C was invented in 1970s but it is still relevant even after 50+ years.
I have learned C since 2009 and I am still working with C codes on a day-to-day life. C will still go further in the coming years as it is one of the widely used high level language that works with low level software layer that deals with the hardware programming.
If you understand the C it will also help you in understanding how the system or Operating System works. Once you know these things it can give you a good job in a very reputed companies like Intel, AMD, Cisco, Qualcomm, Broadcom, etc.
C Programming is fun if you are interested in it. And, if you are interested, I am ready to spend as much time as you ask in explaining everything you need to understand.