Prime number Program in C is a very interesting case that give you the opportunity to learn logic building before you start programming.
Most other websites just give you the same program but in this article I will of course explain the program but also explain some more cases which I though but it does not work.
It is important to work with negative or non-working cases so that you will understand the working logic very strongly.
Finding the logic for Prime Number C Program
Before discussing on the logic we need to first understand what is a prime number.
A number which is only divisible by 1 or by itself is called as a Prime Number. Simple!
But how do you do that in C Programming?
To be able to find a Prime number in C Language, we will have to handle many cases.
A number given by the user can be 0, 2, 5, an even number, etc. Based on several cases, we will add different conditions in C to find out if a number is a Prime number or not. Let’s look at the actual logic of the program.
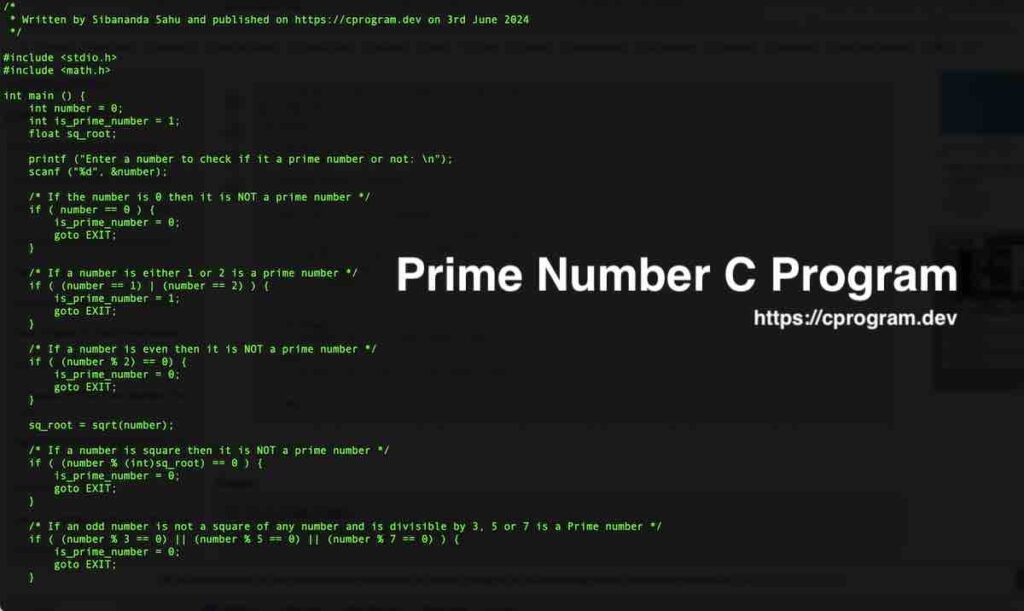
Actual Logic
I have written this logic on my own and this is the most simplest one and different among anything that you find on the internet.
If you find any difficulty in understanding the logic, please leave a comment below and I will respond to you as soon as possible.
The basic principle we use is, if a number is NOT a prime number, then it is a prime number. Basically, we handle many different types of conditions where a number could not be a prime number.
So, what all things can I use from C Programming Language to find a given number is prime number or not?
- The very first thing is that we need to test the same number by dividing it by 2, 3, 5 etc. to know if it is a unique number or divisible by other number(s) or not. Which means, we need to test the given number with several conditional cases as below:
- By default 1 is a prime number.
- Then, 2 is a Prime Number.
- Except 2 any other even number is NOT a Prime number.
- Now comes only how to find an odd number as a prime number or not!
- Initially I thought if we just loop through to divide the number by any number from 3 to 9 we will get the prime number. but I was wrong!
- Let’s take a look on few examples. Most of the numbers like 12, 15, 21, 25, 49, and so on.. are directly divisible by 3, 5 and 7.
- But let’s take an example of 11’s square, or may be 13’s square. The numbers are 121, 269 etc. are only divisible by 11 and 13 respectively but they are not divisible by 3, 5 or 7.
- So, I decided to go with a different approach then others to find a Prime number Program in C.
- We will find the square root of a number. If the user given number is divisible by the square root we found, then the number is not a prime number.
- Basically any number that is left out is a Prime number.
Writing the Final Prime Number Program in C
To be able to understand this problem you must already know the below things in C:
- C datatypes
- C Conditional statement (if else or switch case)
- logical or condition (represent by the symbol ‘||’ in C)
- modulo operator ‘%’ in C
- label and goto instruction in c
- find square root of a number by using sqrt() library function from math.h
/*
* Written by Sibananda Sahu and published on https://cprogram.dev on 3rd June 2024
*/
#include <stdio.h>
#include <math.h>
int main () {
int number = 0;
int is_prime_number = 1;
float sq_root;
printf ("Enter a number to check if it a prime number or not: \n");
scanf ("%d", &number);
/* If the number is 0 then it is NOT a prime number */
if ( number == 0 ) {
is_prime_number = 0;
goto EXIT;
}
/* If a number is either 1, 2, 3, 5 or 7 is a prime number */
switch ( number) {
case 1:
case 2:
case 3:
case 5:
case 7:
is_prime_number = 1;
goto EXIT;
/* Break is not needed as we are using goto just before this */
// break;
default:
break;
}
/* If a number is an even number then it is NOT a prime number */
if ( (number % 2) == 0) {
is_prime_number = 0;
goto EXIT;
}
sq_root = sqrt(number);
/* If a number is square then it is NOT a prime number */
if ( (number % (int)sq_root) == 0 ) {
is_prime_number = 0;
goto EXIT;
}
/* If an odd number is not a square of any number and is divisible by 3, 5 or 7 is a Prime number */
if ( (number % 3 == 0) || (number % 5 == 0) || (number % 7 == 0) ) {
is_prime_number = 0;
goto EXIT;
}
EXIT:
if ( is_prime_number == 1 ) {
printf ( "The number %d is a Prime Number.\n", number );
} else {
printf ( "The number %d is NOT a Prime Number.\n", number );
}
return 0;
}
Code language: PHP (php)
I have got the below output for the above Prime C program:
$ ./a.out
Enter a number to check if it a prime number or not:
5
The number 5 is a Prime Number.
$ ./a.out
Enter a number to check if it a prime number or not:
7
The number 7 is a Prime Number.
$ ./a.out
Enter a number to check if it a prime number or not:
9
The number 9 is NOT a Prime Number.
$ ./a.out
Enter a number to check if it a prime number or not:
11
The number 11 is a Prime Number.
$ ./a.out
Enter a number to check if it a prime number or not:
4
The number 4 is NOT a Prime Number.
$ ./a.out
Enter a number to check if it a prime number or not:
121
The number 121 is NOT a Prime Number.
Code language: JavaScript (javascript)
Conclusion
When I searched on Google about the solution I found almost the same answer across many websites. In fact, I found it strange that how people not consider about explaining to beginners except a very few websites.
I always thought to keep a simple logic to find the prime number Program in C and that’s where I created a different solution to the problem which is simple and a thoughtful answer.
I have tested several numbers and it worked pretty well. If you find any corner case that does not work, please leave that case in the comment I will fix the issue.