When you write the leap year program in C you will understand about converting mathematical requirement into code logic.
It is important first to understand what a leap year is, it is then only we will be able to write a C program on it.
Let’s take a look.
Understanding a Leap Year
In a year where we all think of having 365 days, the earth’s year is actually 365 days, 5 hours, 48 minutes, and 46 seconds long.
Which is 11 minutes 14 seconds less than 6 hours in a year.
Which means, in 4 years, we will have an extra 23 hours, 15 minutes and 04 seconds, not really a complete 24 hours but still we see it as an extra day in 4 years.
But the short of approximate ~11 minutes in a year will make a short of ~1440 minutes (which is a day) in approximately ~131 years.
This is the reason we skip a leap year every century (100 years) and so almost 3 leap year days are skipped every ~400 years.
To make it clear, let’s understand this way. When the year 1600 is a leap year, then 1700, 1800, 1900 will NOT be leap years and 2000 will again become a leap year.
Understanding the Logic for Leap year C Program
Now let’s go to build a pseudo code before we actually write the C program.
Actual Logic
We will have to build some logic to rule out which numbers are not leap years. Then whichever is left out, lets see which is a leap year.
Based on the above understanding of a Leap Year, this is how we will do the same in C Program:
- A year or a number which is divisible by 4 is generally a leap year.
- In addition, a number which is divisible by 4 but also divisible by 400 is a leap year.
- Yet again, a number which is divisible by 4 and also divisible by 100, is NOT a leap year.
Which means, any number that is divisible by 4 may NOT be a leap year. We still need to check further.
Which also means, any number that is divisible by 400 is straight forward a leap year.
But any number that is divisible by 4 but also divisible by 100 may not be a leap year.
Writing the Leap Year Program in C
To be able to understand this problem you must already know the below things in C:
- C datatypes
- C Conditional statement (if else or switch case)
- logical or condition (represent by the symbol ‘||’ in C)
- modulo operator ‘%’ in C
/*
* Leap year program written by Sibananda Sahu
* published on https://cprogram.dev on 4th June 2024
* Last updated on 4th June 2024
*/
#include <stdio.h>
int main () {
int year = 0;
int is_leap_year = 1;
printf ("Enter a number to check if it is a Leap Year or not: \n");
scanf ("%d", &year);
/* If the number is divisible by 4, there is a chance that it is a leap year */
if ( year % 4 == 0 ) {
/* A number divisible by 4 and 400 is a leap year */
if ( year % 400 == 0 ) {
printf ("Year %d is a LEAP YEAR\n", year);
}
/* A number divisible by 4 and 100 is NOT a leap year */
else if ( year % 100 == 0 ) {
printf ("Year %d is a NOT a leap year\n", year);
}
/* A number not divisible by 400 nor 100, but we already know it is divisible by 4 is a leap year */
else {
printf ("Year %d is a LEAP YEAR\n", year);
}
} // %4 if ends here but we have an else case to it
/* A number that is not divisible by 4 is NOT a leap year. */
else {
printf ("Year %d is a NOT a leap year\n", year);
} // %4 if else ends here
return 0;
}
Code language: PHP (php)
The same program can also be written as below:
/*
* Leap year program written by Sibananda Sahu
* published on https://cprogram.dev on 4th June 2024
* Last updated on 4th June 2024
*/
#include <stdio.h>
int main () {
int year = 0;
int is_leap_year = 1;
printf ("Enter a number to check if it is a Leap Year or not: \n");
scanf ("%d", &year);
/* If the number is divisible by 400, is a leap year */
if ( year % 400 == 0 ) {
printf ("Year %d is a LEAP YEAR\n", year);
}
/* A number NOT divisible by 400 and divisible by 100 is NOT a leap year */
else if ( year % 100 == 0 ) {
printf ("Year %d is a NOT a leap year\n", year);
}
/* A number not divisible by 400 nor 100, but divisible by 4 is a leap year */
else if ( year % 4 == 0 ) {
printf ("Year %d is a LEAP YEAR\n", year);
}
/* A number that is not divisible by 4 is NOT a leap year. */
else {
printf ("Year %d is a NOT a leap year\n", year);
} // %400 if else ends here
return 0;
}
Code language: PHP (php)
Either of the program has the exact same logic but in two different forms and both of them work.
Output
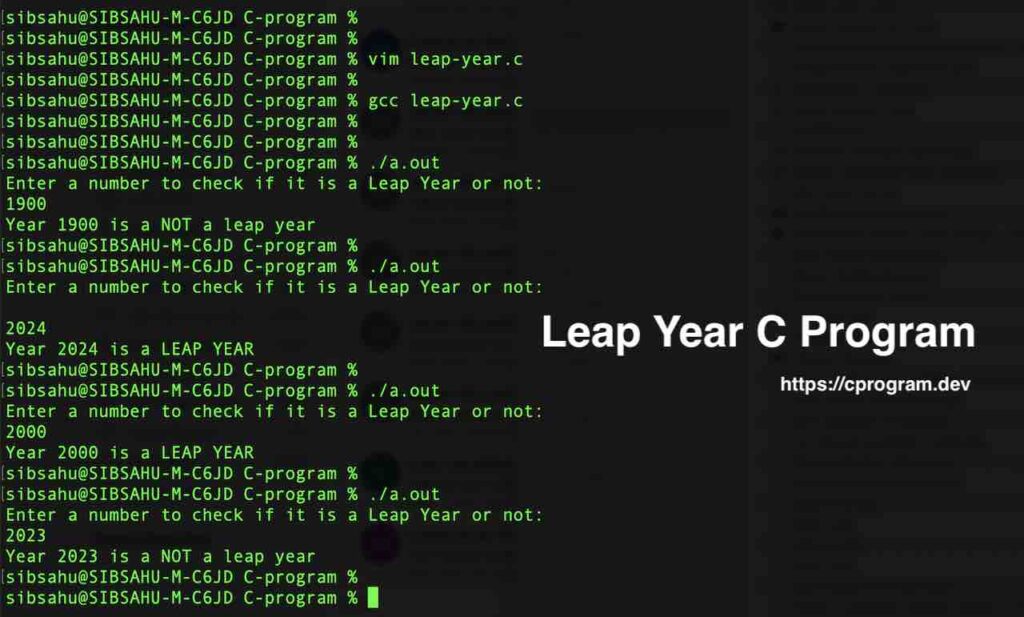
$ ./a.out
Enter a number to check if it is a Leap Year or not:
1900
Year 1900 is a NOT a leap year
$ ./a.out
Enter a number to check if it is a Leap Year or not:
2024
Year 2024 is a LEAP YEAR
$ ./a.out
Enter a number to check if it is a Leap Year or not:
2000
Year 2000 is a LEAP YEAR
$ ./a.out
Enter a number to check if it is a Leap Year or not:
2023
Year 2023 is a NOT a leap year
Conclusion
After completing the Leap Year C Program you will understand nested if else or if else in a better way. In addition, you will also understand using the modulo ‘%’ operator.
Understanding the real conditions before writing a program is necessary. Once you understand the actual mathematics then you may need to find which condition to keep and which one to rule out while building the program.
I have tested this Leap Year Program in C with several numbers and it worked pretty well. If you find any corner case that does not work, please leave that case in the comment I will fix the issue.